Set of pictures edited using Photoshop and Python's OpenCV library.
My goal was to enhance interest by adding colored blocks, resulting in a surprising effect.
The images were slightly edited in Photoshop and then Python codes were applied.
The images were slightly edited in Photoshop and then Python codes were applied.
I chose to apply codes with random parameters (like the size of the block and its location in the image) and controlled parameters (for example - the color of the blocks is determined by RGB code).
import cv2
import random as r
import numpy as np
from matplotlib import pyplot as plt
import random as r
import numpy as np
from matplotlib import pyplot as plt
input_image = cv2.imread("/Users/Noa/Documents/ME/EDITS/edit/DSC03559A.jpg")
image = cv2.cvtColor(input_image, cv2.COLOR_BGR2RGB)
plt.imshow(image)
plt.imshow(image)
height = image.shape[0]
width = image.shape[1]
width = image.shape[1]
overlay = image.copy()
x = r.randint(0,width)
y = r.randint(0,height)
w = width
h = height
size1 = r.randint(200,600)
size2 = r.randint(200,1000)
size2 = r.randint(200,1000)
y2 = r.randint(0,height)
y3 = r.randint(0,height)
y3 = r.randint(0,height)
# Rectangles
cv2.rectangle(overlay, (0, y), (w, y+size2), (243, 203, 242), -1)
cv2.rectangle(overlay, (0, y), (w, y+size1), (245, 143, 35), -1)
cv2.rectangle(overlay, (x, 0), (x+size2, h), (95, 30, 194), -1)
cv2.rectangle(overlay, (0, y), (w, y+size2), (243, 203, 242), -1)
cv2.rectangle(overlay, (0, y), (w, y+size1), (245, 143, 35), -1)
cv2.rectangle(overlay, (x, 0), (x+size2, h), (95, 30, 194), -1)
# Transparency value
alpha = 0.70
alpha = 0.70
# Perform weighted addition of the input image and the overlay
result = cv2.addWeighted(overlay, alpha, image, 1 - alpha, 0)
result = cv2.addWeighted(overlay, alpha, image, 1 - alpha, 0)
cv2.imshow('result', result)
cv2.waitKey()
fresult = cv2.cvtColor(result, cv2.COLOR_RGB2BGR)
cv2.imwrite('C:/Users/Noa/Documents/ME/EDITS/edit/CODE/img17.jpg',fresult)
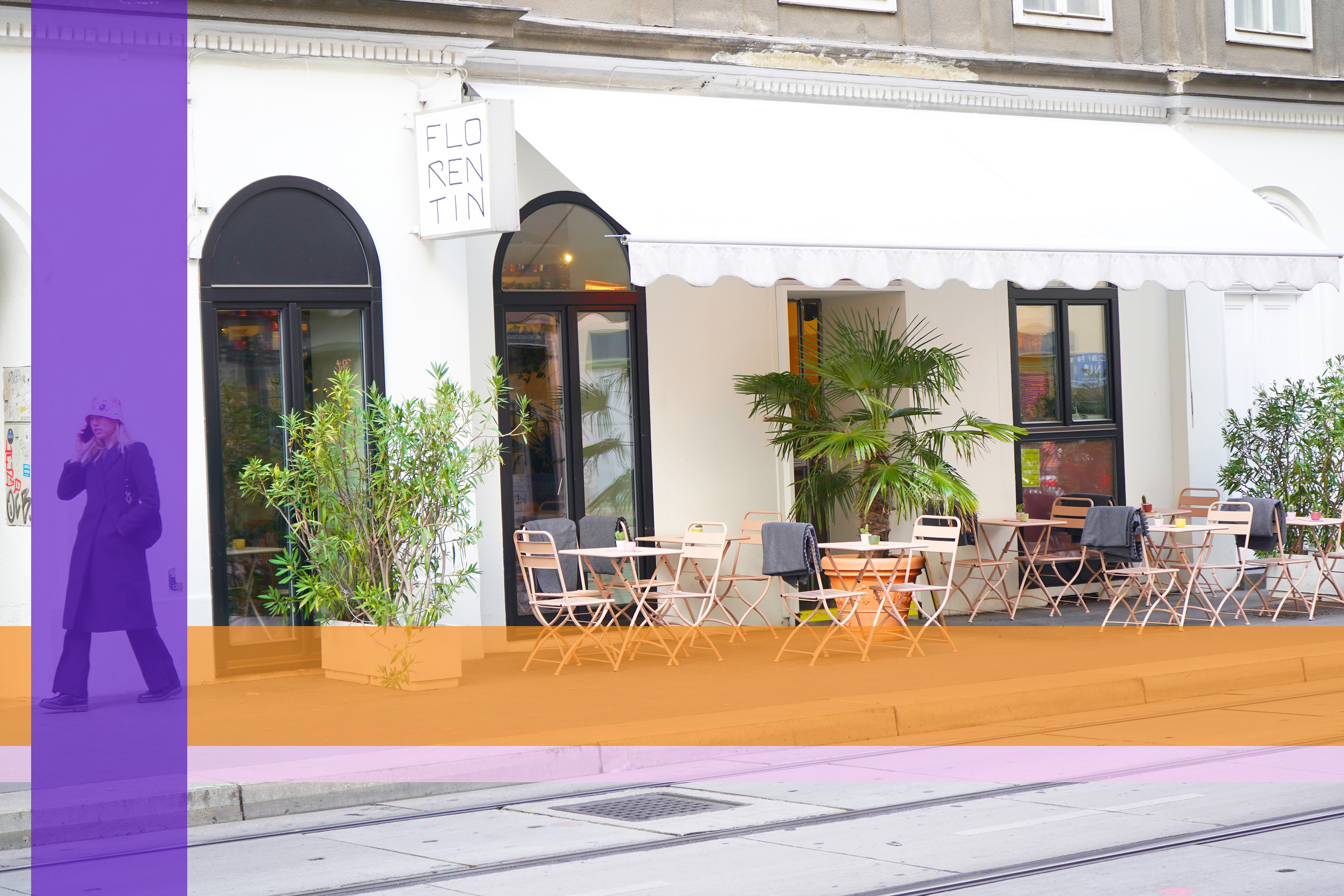
import cv2
import random as r
import numpy as np
from matplotlib import pyplot as plt
import random as r
import numpy as np
from matplotlib import pyplot as plt
input_image = cv2.imread("/Users/Noa/Documents/ME/EDITS/edit/DSC03530A.jpg")
image = cv2.cvtColor(input_image, cv2.COLOR_BGR2RGB)
plt.imshow(image)
image = cv2.cvtColor(input_image, cv2.COLOR_BGR2RGB)
plt.imshow(image)
height = image.shape[0]
width = image.shape[1]
width = image.shape[1]
overlay = image.copy()
x = r.randint(0,width)
y = r.randint(0,height)
w = width
h = height
size1 = r.randint(200,600)
size2 = r.randint(200,1000)
size2 = r.randint(200,1000)
size3 = r.randint(200,600)
size4 = r.randint(100,400)
size4 = r.randint(100,400)
y1 = r.randint(0,height)
y2 = r.randint(0,height)
y2 = r.randint(0,height)
# Rectangles
cv2.rectangle(overlay, (0, y), (w, y+size1), (4, 39, 115), -1)
cv2.rectangle(overlay, (x, 0), (x+size2, h), (241, 18, 235), -1)
# Transparency value
alpha = 0.70
# Perform weighted addition of the input image and the overlay
result = cv2.addWeighted(overlay, alpha, image, 1 - alpha, 0)
result = cv2.addWeighted(overlay, alpha, image, 1 - alpha, 0)
fresult = cv2.cvtColor(result, cv2.COLOR_RGB2BGR)
plt.imshow(fresult)
cv2.imwrite('C:/Users/Noa/Documents/ME/EDITS/edit/CODE/img15.jpg',fresult)
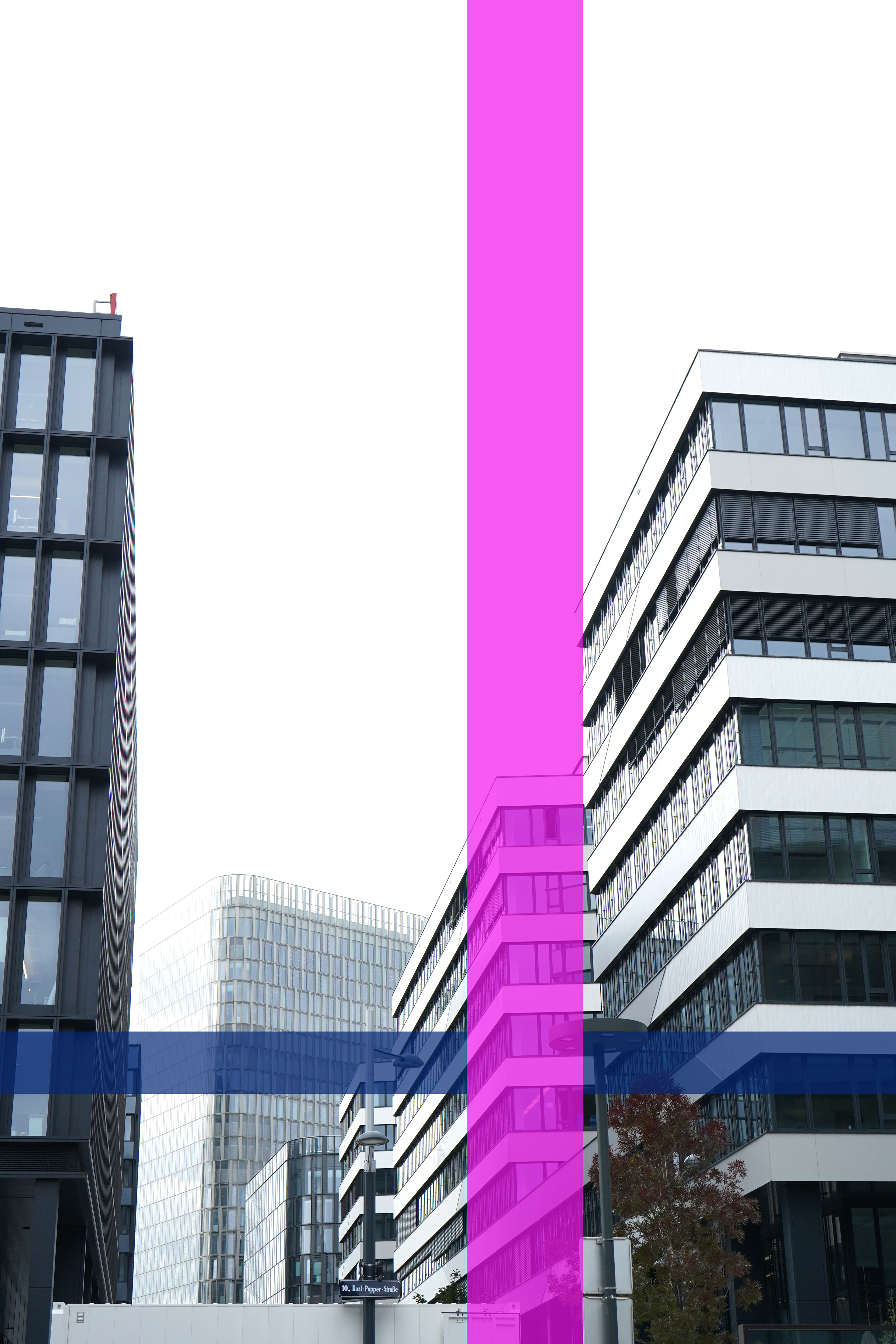
The combination of controlled and random parameters results in highlighting features we would not have focused on if all the parameters had been controlled.